Top SQL Concepts
Core SQL concepts are vital for efficiently managing and manipulating relational databases, ensuring data accuracy, and optimizing performance.
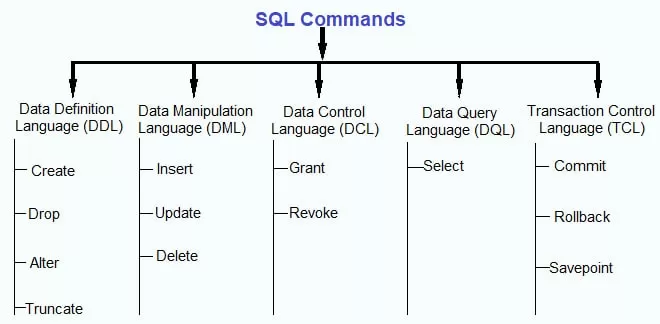
1. SQL Introduction:
SQL, or Structured Query Language, is the standard language for managing and manipulating relational databases. It enables users to perform CRUD operations (Create, Read, Update, Delete) on database data, making it crucial for effective database management.
2. Databases and Tables:
- Database: A systematically organized collection of data stored in a computer, typically structured in tables.
- Table: A set of data organized into rows and columns, with each row representing a record and each column representing a field within that record.
3. Data Types:
- Numeric Data Types: Include INTEGER, FLOAT, DECIMAL, etc.
- Character Data Types: Include CHAR, VARCHAR, TEXT, etc.
- Date and Time Data Types: Include DATE, TIME, DATETIME, etc.
- Boolean Data Type: BOOLEAN.
4. Core SQL Commands:
- SELECT: Retrieves data from a database.
- INSERT: Adds new data into a table.
- UPDATE: Modifies existing data in a table.
- DELETE: Removes data from a table.
5. SQL Clauses:
- WHERE: Filters records based on specified conditions.
- ORDER BY: Sorts the result set in either ascending (ASC) or descending (DESC) order.
- GROUP BY: Groups rows with the same values in specified columns into summary rows.
- HAVING: Filters groups based on specified conditions, usually used with GROUP BY.
6. JOIN Operations:
- INNER JOIN: Selects records with matching values in both tables.
- LEFT JOIN (LEFT OUTER JOIN): Selects all records from the left table and the matched records from the right table.
- RIGHT JOIN (RIGHT OUTER JOIN): Selects all records from the right table and the matched records from the left table.
- FULL JOIN (FULL OUTER JOIN): Selects all records when there is a match in either left or right table.
7. Aggregate Functions:
- COUNT(): Returns the number of rows that match a specified condition.
- SUM(): Returns the total sum of a numeric column.
- AVG(): Returns the average value of a numeric column.
- MAX(): Returns the maximum value in a column.
- MIN(): Returns the minimum value in a column.
8. Subqueries:
A subquery is a query embedded inside another query. It can be used in SELECT, INSERT, UPDATE, or DELETE statements or within another subquery. Subqueries can return single values or lists of records.
9. Indexing:
Indexes enhance data retrieval speed by creating a pointer to quickly locate data in a database table. Indexes can be created on one or more columns.
10. SQL Constraints:
Constraints enforce rules on data columns in a table to ensure data accuracy and reliability. Common constraints include:
- NOT NULL: Ensures that a column cannot have a NULL value.
- UNIQUE: Ensures that all values in a column are unique.
- PRIMARY KEY: Combines NOT NULL and UNIQUE. Uniquely identifies each row in a table.
- FOREIGN KEY: Ensures referential integrity between tables.
- CHECK: Ensures all values in a column satisfy a specific condition.
11. Transactions:
A transaction is a series of one or more SQL statements executed as a single unit, ensuring data integrity. Key elements include:
- BEGIN TRANSACTION: Starts a transaction.
- COMMIT: Permanently saves the transaction changes.
- ROLLBACK: Reverts the changes made by the transaction.
12. SQL Functions:
SQL includes various built-in functions for data operations:
- String Functions: e.g., CONCAT(), SUBSTR(), LENGTH().
- Numeric Functions: e.g., ROUND(), CEIL(), FLOOR().
- Date Functions: e.g., NOW(), CURDATE(), DATEADD().
13. Views:
A view is a virtual table based on the result of an SQL query. Views simplify complex queries and enhance security by restricting access to specific data.
14. Stored Procedures and Triggers:
- Stored Procedures: A set of SQL statements with a name that can be executed as a unit.
- Triggers: SQL code that automatically executes in response to specific events on a table or view.
15. Normalization:
Normalization organizes database data to minimize redundancy and improve integrity. This involves dividing large tables into smaller ones and defining relationships between them.
What's Your Reaction?
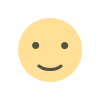
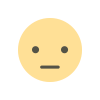
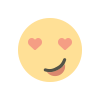
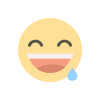
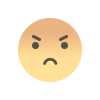
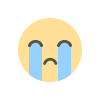
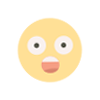