Java List Interface: Key Features, Methods, and Usage Scenarios
The List interface in Java is a versatile and essential tool for working with ordered collections of elements. With implementations like ArrayList, LinkedList, and Vector, it offers various performance characteristics suited to different use cases. The List interface’s methods enable developers to efficiently add, remove, and access elements, making it a vital component of the Java Collections Framework. Whether you're storing data, implementing caching mechanisms, or performing complex manipulations, the List interface offers the necessary functionality to manage collections effectively.
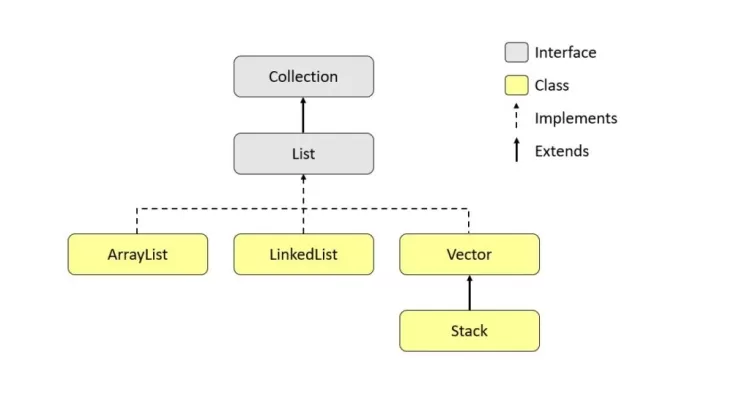
The "List interface" in Java is a core element of the Java Collections Framework, designed to represent ordered collections of elements. As a subinterface of the Collection interface, the List interface includes methods for handling lists that allow duplicate entries and provide index-based access to elements. This makes it a crucial tool for developers working with sequential collections of objects.
Key Characteristics:
1. Order: Lists preserve the order of elements as they are inserted, which means each element’s position is maintained, allowing developers to access them in the sequence they were added.
2. Duplicates: Lists can contain duplicate elements. This feature is useful when both the order and frequency of elements are significant, such as in a list of customer orders or a sequence of events.
3. Index-based Access: Elements in a list can be accessed through their integer index, offering a direct way to retrieve, insert, or remove elements at specific positions.
Common Implementations:
Several concrete classes implement the List interface, each providing unique performance characteristics and suitable for different scenarios. Some of the popular implementations include:
1. ArrayList: This resizable array implementation of the List interface offers fast random access to elements due to its array backing. However, insertions and deletions, especially in the middle, can be slow as they may require shifting elements. ArrayList is ideal for scenarios with more read operations than write operations.
List
2. LinkedList: A doubly-linked list implementation of the List and Deque interfaces, it offers quick insertions and deletions since elements do not need to be shifted. However, random access is slower compared to ArrayList due to the need for traversal from the list’s beginning. LinkedList is suitable for applications with frequent additions and removals.
List
3. Vector: This synchronized version of ArrayList ensures thread-safe operations but introduces synchronization overhead, making it less efficient. Vector is not commonly used in modern applications due to the availability of more efficient alternatives like ArrayList and concurrent collections.
List
Important Methods:
The List interface offers several methods that enable developers to manipulate and interact with elements within a list. Some key methods include:
add(E e): Appends the specified element to the list’s end.
list.add("Mango");
add(int index, E element): Inserts the specified element at a specified position in the list.
list.add(1, "Orange");
get(int index): Retrieves the element at the specified position in the list.
String fruit = list.get(1); // Output: Orange
remove(int index): Removes the element at the specified position in the list.
list.remove(0); // Removes "Apple"
set(int index, E element): Replaces the element at a specified position with the specified element.
list.set(0, "Grapes"); // Replaces "Banana" with "Grapes"
size(): Returns the number of elements in the list.
int size = list.size(); // Output: 3
contains(Object o): Checks if the list contains the specified element.
boolean contains = list.contains("Cherry"); // Output: true
indexOf(Object o): Finds the index of the first occurrence of the specified element, or returns -1 if it’s not found.
int index = list.indexOf("Cherry"); // Output: 2
- subList(int fromIndex, int toIndex): Provides a view of the list portion between the specified fromIndex (inclusive) and toIndex (exclusive).
Listsublist = list.subList(0, 2); // Output: ["Grapes", "Orange"]
Usage Scenarios:
1. Storing and Manipulating Data: Lists are extensively used for storing and manipulating groups of objects across various applications. For instance, an ArrayList can store a dynamic list of items in a shopping cart, where item order matters.
List
2. Searching and Sorting: Lists can be searched and sorted using algorithms from the Collections framework. For example, the Collections.sort method sorts elements in a list.
Collections.sort(shoppingCart); // Sorts the shopping cart items in ascending order
3. Caching: Lists such as ArrayList and LinkedList can be used to implement caching mechanisms, storing recently accessed items for quick retrieval.
List
4. Event Handling: Lists like LinkedList are often used to manage event listeners in graphical user interfaces, allowing efficient addition and removal of listeners.
List
5. Data Structures: Specialized lists like PriorityQueue and TreeSet (related but not direct implementations of List) are used to create advanced data structures such as priority queues and sorted sets.
PriorityQueue
What's Your Reaction?
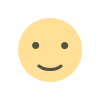
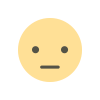
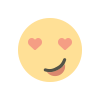
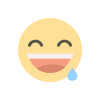
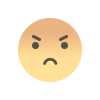
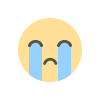
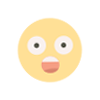