Java Streams: Transforming Data Processing Methods
Java Streams, introduced in Java 8, offer a robust abstraction for processing sequences of elements in a functional manner. They provide a concise and expressive method for managing data collections, improving code readability and enabling parallel processing to boost performance.
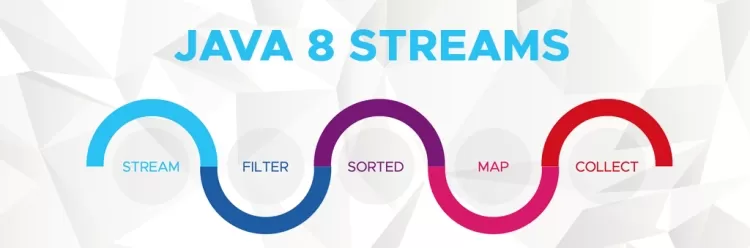
What are Java Streams?
A Stream in Java represents a sequence of elements that can be processed in parallel or sequentially. Unlike collections, streams do not store elements; instead, they convey elements from a source (such as a collection, array, or I/O channel) through a pipeline of computational operations. This abstraction allows for concise and readable code, focusing on the "what" rather than the "how" of data processing.
Key Characteristics
-
Pipelining: Streams support pipelined operations, enabling multiple operations to be chained together to form a processing pipeline. This allows for fluent processing of data in a single pass, improving efficiency.
-
Internal Iteration: Streams handle the iteration of elements internally, abstracting away the need for external loops. This reduces boilerplate code and minimizes the risk of errors.
-
Laziness: Streams are lazy by nature, meaning that intermediate operations are not executed until a terminal operation is invoked. This can lead to significant performance improvements by avoiding unnecessary computations.
Stream Operations
Stream operations are divided into two main categories:
-
Intermediate Operations: These operations transform a stream into another stream. They are lazy and only executed when a terminal operation is called. Common intermediate operations include:
-
filter(Predicate
) : Filters elements based on a given condition.List
Output:[Alice]
-
map(Function
) : Transforms each element using a provided function.List
->n * n) .collect(Collectors.toList()); Output: [1, 4, 9]
-
sorted(): Sorts the elements in natural order or using a custom comparator.
List
"Cherry");List
.sorted().collect(Collectors.toList()); Output: [Apple, Banana,
Cherry]
-
-
Terminal Operations: These operations produce a result or a side-effect and terminate the stream pipeline. Common terminal operations include:
-
collect(Collector
) : Collects elements into a collection, such as a List, Set, or Map.List
"Jane","Jack"); Set
Jack] -
forEach(Consumer
) : Performs an action for each element in the stream.List
.forEach(System.out::println);Output: Apple Banana Cherry
-
reduce(BinaryOperator
) : Performs a reduction on the elements, such as summing them up.List
Integer::sum);Output: 15
-
Creating Streams
There are several ways to create streams in Java:
-
From Collections:
List
-
From Arrays:
String[] array = {"Apple", "Banana", "Cherry"}; Stream
-
Using Stream.of():
Stream
-
Generating Streams:
Stream
-
Creating Infinite Streams:
Stream
Advanced Stream Features
-
Parallel Streams: Streams can be easily converted to parallel streams to leverage multi-core processors. Parallel streams divide the stream into multiple sub-streams and process them in parallel, improving performance for large datasets.
List
-
Custom Collectors: The
Collectors
utility class provides several predefined collectors, but custom collectors can also be created to handle specific collection requirements.Collector?, List
> toUpperCaseList = Collectors.mapping(String::toUpperCase, Collectors.toList()); List.collect(toUpperCaseList); Output: [A, B, C]
Use Cases
-
Data Transformation: Java Streams are ideal for transforming data from one form to another, such as filtering, mapping, and reducing collections.
-
Batch Processing: Streams provide a powerful mechanism for processing large data sets in batches, enabling efficient data handling.
-
Event Handling: Streams can be used to process sequences of events, applying transformations and filters as needed.
-
Aggregation: Streams simplify the process of aggregating data, such as summing, averaging, and counting elements.
Java Streams offer a powerful and flexible way to process data in a declarative manner. They allow developers to write clean, readable, and efficient code for handling collections and other data sources. By leveraging streams, developers can perform complex data processing tasks with ease, making Java Streams an essential tool for modern Java development.
What's Your Reaction?
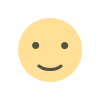
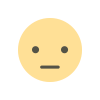
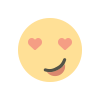
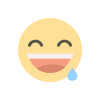
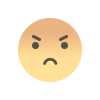
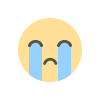
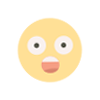