Java Multithreading
Java's multithreading capabilities offer a robust framework for concurrent programming, significantly boosting application performance and resource utilization. With features such as thread synchronization, the Executor framework, and diverse thread management techniques, Java empowers developers to craft efficient, responsive, and high-performing applications. Mastering and effectively applying multithreading techniques is essential for developing scalable and responsive software solutions.
Key Concepts of Multithreading
-
Thread: A thread is a single path of execution within a program. Each thread runs independently, and multiple threads can run concurrently within a single program.
-
Process vs. Thread: A process is a self-contained execution environment, while a thread is a smaller unit of execution within a process. Threads share the same memory space, while processes have separate memory spaces.
-
Concurrency vs. Parallelism: Concurrency is the ability to run multiple threads simultaneously, while parallelism involves executing multiple threads on different processors or cores. Java supports both concurrency and parallelism.
Creating Threads in Java
There are two primary ways to create threads in Java:
-
Implementing the Runnable Interface:
-
This approach involves implementing the Runnable interface and defining the run() method.
-
Example:
class MyRunnable implements Runnable { public void run() { System.out.println("Thread is running"); } } public class Main { public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread thread = new Thread(myRunnable); thread.start(); } }
-
-
Extending the Thread Class:
-
This approach involves extending the Thread class and overriding the run() method.
-
Example:
class MyThread extends Thread { public void run() { System.out.println("Thread is running"); } } public class Main { public static void main(String[] args) { MyThread myThread = new MyThread(); myThread.start(); } }
-
Thread Lifecycle
A thread goes through several stages in its lifecycle:
-
New: A new thread is created but not yet started.
-
Runnable: The thread is ready to run and waiting for CPU time.
-
Running: The thread is actively executing its task.
-
Waiting: The thread is waiting for a resource or condition to be satisfied.
-
Terminated: The thread has completed its execution.
Thread Synchronization
Thread synchronization is crucial in multithreading to prevent race conditions and ensure thread safety. Java provides several mechanisms for synchronizing threads:
-
synchronized Method: This ensures that only one thread can execute a synchronized method at a time.
-
Example:
class Counter { private int count = 0; public synchronized void increment() { count++; } public int getCount() { return count; } } public class Main { public static void main(String[] args) { Counter counter = new Counter(); Runnable task = () -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }; Thread thread1 = new Thread(task); Thread thread2 = new Thread(task); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Count: " + counter.getCount()); } }
-
-
synchronized Block: This allows more fine-grained control over synchronization by synchronizing only a block of code.
-
Example:
class Counter { private int count = 0; public void increment() { synchronized(this) { count++; } } public int getCount() { return count; } }
-
-
Lock Interface: Java provides the Lock interface from the java.util.concurrent.locks package for advanced synchronization.
-
Example:
import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; class Counter { private int count = 0; private Lock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } public int getCount() { return count; } } public class Main { public static void main(String[] args) { Counter counter = new Counter(); Runnable task = () -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }; Thread thread1 = new Thread(task); Thread thread2 = new Thread(task); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Count: " + counter.getCount()); } }
-
Executor Framework
The Executor framework in java.util.concurrent provides a higher-level replacement for managing threads. It decouples task submission from task execution, allowing better management of thread pools.
-
Example using ExecutorService:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class Main { public static void main(String[] args) { ExecutorService executorService = Executors.newFixedThreadPool(2); Runnable task1 = () -> System.out.println("Task 1 is running"); Runnable task2 = () -> System.out.println("Task 2 is running"); executorService.submit(task1); executorService.submit(task2); executorService.shutdown(); } }
Multithreading in Java provides a robust framework for concurrent programming, improving application performance and resource utilization. With features like thread synchronization, the Executor framework, and various thread management techniques, Java enables developers to create efficient, responsive, and high-performance applications. Understanding and effectively utilizing multithreading is essential for building scalable and responsive software solutions.
What's Your Reaction?
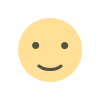
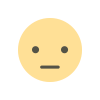
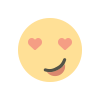
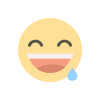
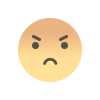
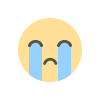
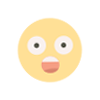