Basic Components of C programming
Tokens, Identifiers, Variables and Data Types are basic building blocks for any programming language. Now let us deep dive into each and every topic
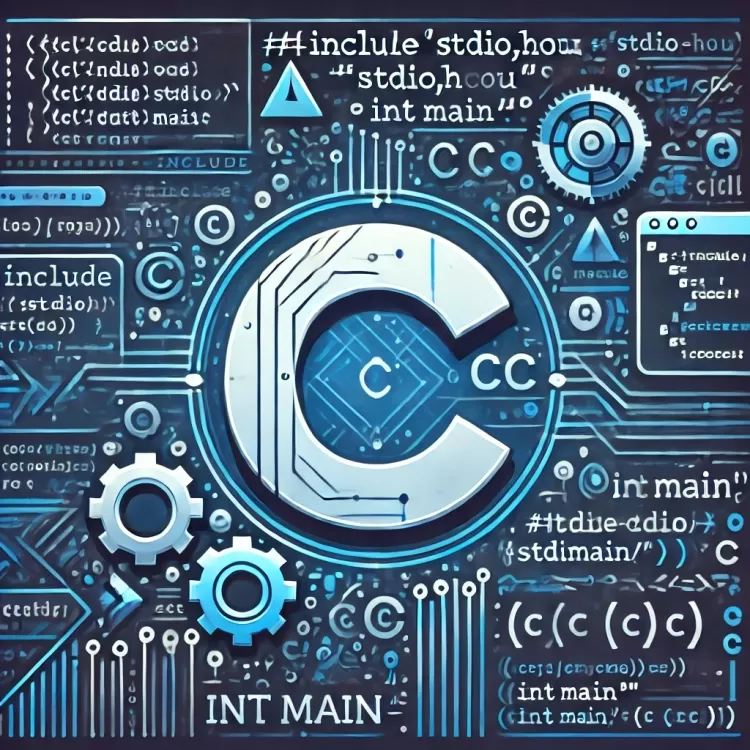
Tokens:
A token in C is the smallest element of programming that is meaningfull to the compiler. For example we cannot create a sentance without using words, similarly we cannot create program in C without using tokens in C. Therefore, we can say that tokens in C is the building block for creating a C programming language.
1.Keywords:
Keywords in C are the reserved words that are pre-defined in C language. Every keyword in C programming have its own functionality. since keywords are predefined words in which compiler uses these word so we cannot use these words as a variable names.If we use these words then compiler will show an error. C language supports 32 keywords given below :
2.Identifiers:
Identifeers in C language are used for naming variables, functions, arrays, structures, etc. Identifers are the user defined words. It can be composed of Uppercase letters, lower case letters, underscore and digits, but starting letter should not start with digit or any special characters.below are given rules for constructiong identifers:
- In Identiferes both uppercase and lowercase letter are different. Therefore, we can say that identifiers are case sensitive.
- First character of an Identifer should be either an alphabet or an underscore and then it can be followed of the character, digit or underscore.
- Keywords cannot be represented as identifers
- The length of the identifer should not exeed 31 characters.
- Commas or blank spaces cannot be specified within an identifer.
- Identifer should be written in such a way that is meaningful and easy to read
3.Constants:
A constant is a value assigned to the variable which will remain the same throughout the program that is constant value cannot be changed. There are 2 ways we can define constant:
- Using const keyword
- Using #define pre-processor
example:
#include
int main() {
const int age = 25; // Integer constant
printf("Age: %d\n", age);
// age = 30; // This is an error because 'age' is constant
return 0;
}
4. Strings in C:
A string in C is an array of characters which are stored in contiguous memory location and terminated by null character i.e., '\0'. This null character denotes the end of the string. String in C are enclosedwithin double quotes, while characters are enclosed within single quotes. The size of a string is determine by the number of characters contained in that string.
there are different ways that we can describe Strings:
char a[10]="smartlocus";// The compiler allocates 10 bytes to the array
char a[]="smartlocus";// The compiler allocates memory at runtime
char a[10]={'s','m','a','r','t','l','o','c','u','s'};// Representation of string in the form of characters
5. Special Characters:
The some special characters which are used in c language cannot be used for another purpose.they are given below:
- Hash{#} : This is also called pre-processor directive. it used at the beginning of the programming.
- Comma{,}: It is used to separating for more than one statement. we use this in evaluating expression and in functions i.e., separating function parameters. When it is used to evalute expression it is called comma operator.
- curly Bracket{}: It is used in opening and closing of blocks, functions and the entire program.
- square bracket[]: It used in representing Arrays ie., Either it is one dimentional or multidimentional
- Asterisk{*}: It is used to represent pointers. And it also an operator for multiplication operation.
- Tilde{~}: It is used as distructor to free the memory.
6. Operators:
An Operator is a symbol which is used to manipulate data. There are many operations like arithmetic, logical, bitwise, etc.
The following types of operators to perform different types of operations in C programming:
- Arithematic Operator
- Assignment Operator
- Relational Operator
- Logical Operator
- Incremental Operator
- Decremental Operator
- Shortcut Operator
- Ternary Operator
- Type Casting Operator
- Size of Operator
- Comma Operator
- Bitwise Operator
What's Your Reaction?
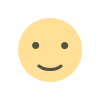
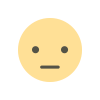
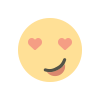
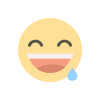
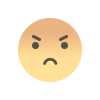
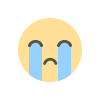
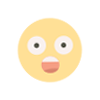