Ternary Operator in C
The ternary operator in C is a shorthand way to write an if-else statement. It is also called the conditional operator and is represented by the symbols ? :. It allows you to evaluate a condition and return one of two values based on whether the condition is true or false.
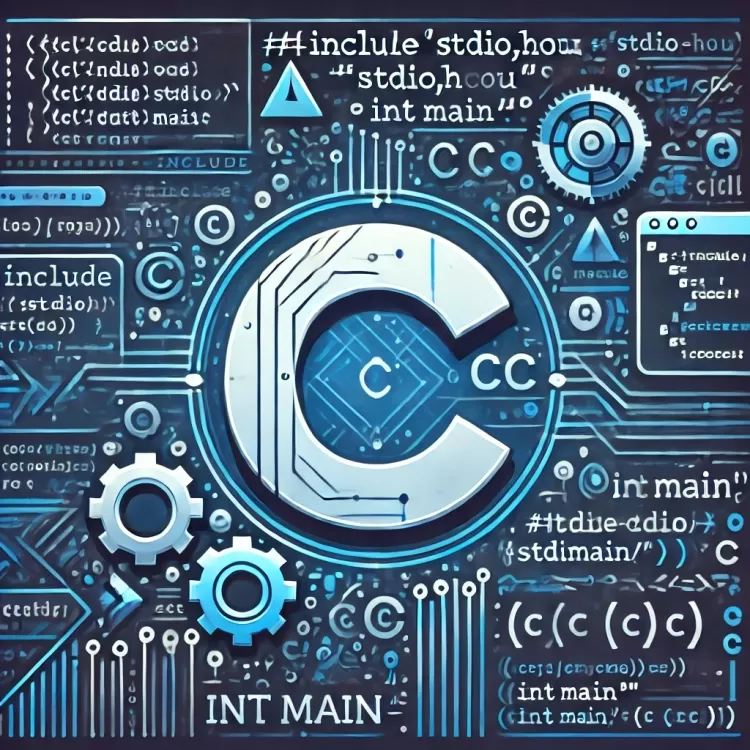
Syntax:
condition ? expression_if_true : expression_if_false;
condition
→ An expression that evaluates to eithertrue
(non-zero) orfalse
(zero).expression_if_true
→ The value or expression that is executed if the condition istrue
.expression_if_false
→ The value or expression that is executed if the condition isfalse
.
Example 1: Basic Usage
#include <stdio.h>
int main() {
int a = 10, b = 20;
int max = (a > b) ? a : b; // If a > b, max = a; otherwise, max = b
printf("Maximum value is: %d\n", max);
return 0;
}
Output:
#include <stdio.h>
int main() {
int num = 7;
(num % 2 == 0) ? printf("Even\n") : printf("Odd\n");
return 0;
}
Output:
Precedence and Associativity:
- Precedence: Lower than arithmetic and relational operators
- Associativity: Right to left
Practice Questions:
- a=10?0?2:3:1; what is the value of a?
- a=2?3?4:5:7?6:8; what is the value of a?
- a=0?7?2:3:4; what is the value of a?
- a=3:2:1?4:6; what is the value of a?
- a=3?2?5:4?6:7:1; what is the value of a?
What's Your Reaction?
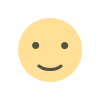
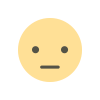
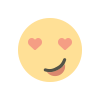
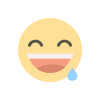
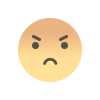
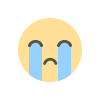
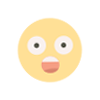