Understanding C Arrays
Arrays in C serve as one of the simplest yet most powerful data structures. They allow you to group similar data items together in a single, contiguous block of memory, making your code more organized and efficient.
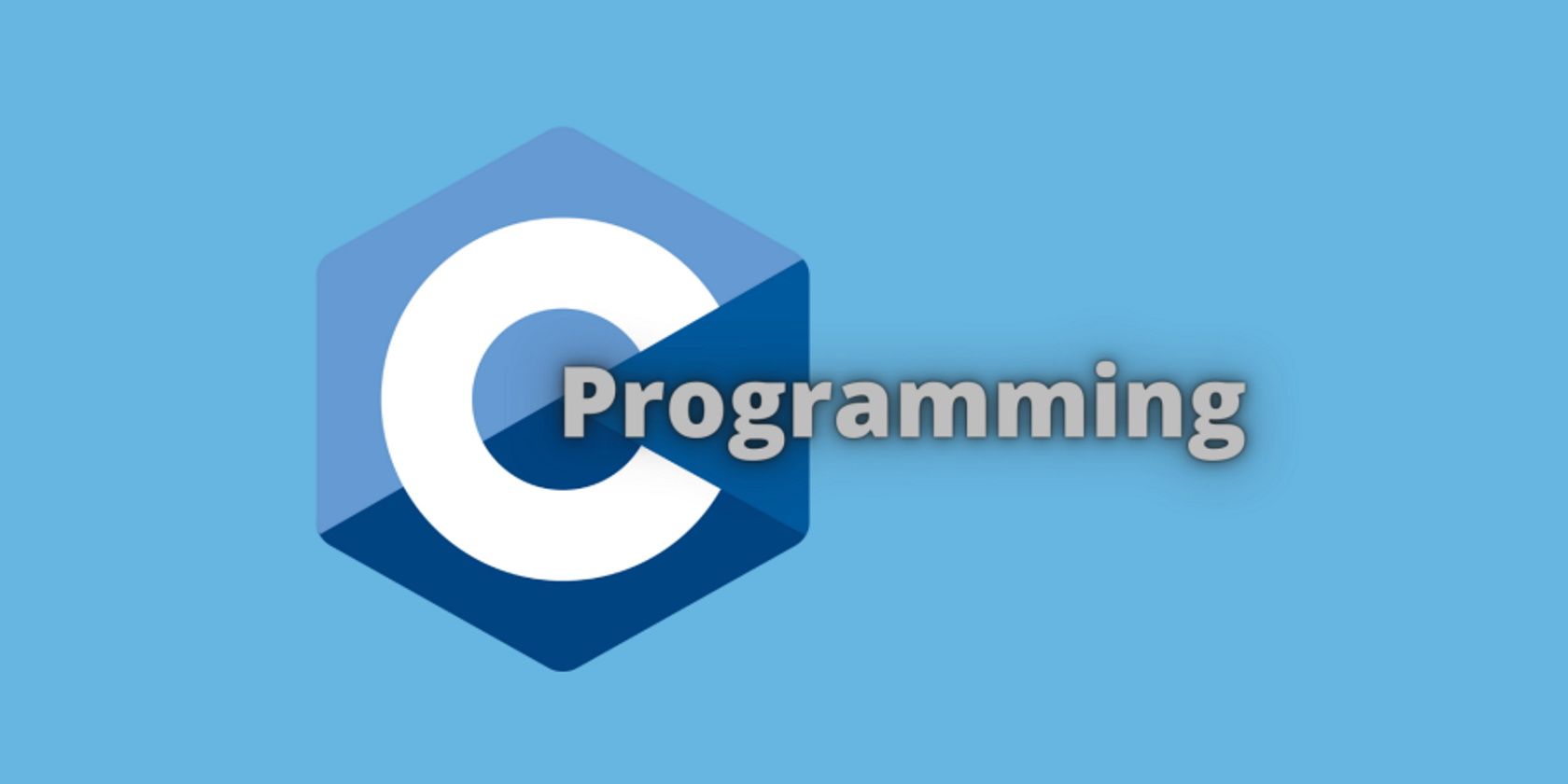
What Is a C Array?
A C array is a collection of elements, all of the same type, stored sequentially in memory. This structure lets you access any element directly via its index. For instance, if you need to store a student's marks for several subjects, an array lets you hold all the marks in one neat package without declaring multiple variables.
Key Properties of C Arrays
- Uniformity: Every element in the array is of the same data type and occupies the same amount of memory.
- Contiguity: The elements are stored in adjacent memory locations, starting from a base address.
- Random Access: Given the base address and the index, you can quickly compute the address of any element, allowing for efficient retrieval.
Benefits of Using Arrays
- Code Efficiency: Arrays simplify the management of multiple similar variables, reducing code redundancy.
- Easy Traversal: With loops like
for
orwhile
, you can effortlessly iterate over all array elements. - Simplified Sorting: Sorting routines can be implemented with minimal code, making data organization straightforward.
- Direct Access: Arrays support random access, so you can fetch any element instantly using its index.
Limitations
- Fixed Size: Once declared, an array's size remains constant. This inflexibility means that arrays cannot adjust dynamically to accommodate more elements, unlike some other data structures.
Declaring Arrays in C
To create an array in C, you define its data type, name, and the number of elements it should hold. The syntax looks like this:
data_type array_name[array_size];
For example:
int marks[5];
In this case, int
specifies the data type, marks
is the name, and 5
is the number of elements the array can hold.
Initializing Arrays
Initialization can be done by assigning values to each element using its index:
marks[0] = 80; // First element
marks[1] = 60;
marks[2] = 70;
marks[3] = 85;
marks[4] = 75;
This method assigns specific values to each position within the array.
Traversing an Array
A common operation with arrays is traversing—visiting each element, often to display or process its value. Here’s a basic program that prints out the elements of the marks
array:
#include <stdio.h>
int main() {
int marks[5] = {80, 60, 70, 85, 75};
for (int i = 0; i < 5; i++) {
printf("%d \n", marks[i]);
}
return 0;
}
This code initializes the array and then uses a loop to print every element.
Declaration with Initialization
C also allows you to declare and initialize an array in one go. For example:
int marks[5] = {20, 30, 40, 50, 60};
If the number of elements is clear from the initialization, you can omit the size:
int marks[] = {20, 30, 40, 50, 60};
Sorting an Array
Sorting arrays is a common task. The following example uses a bubble sort algorithm to arrange elements in descending order:
#include <stdio.h>
void main() {
int a[10] = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
int i, j, temp;
for (i = 0; i < 10; i++) {
for (j = i + 1; j < 10; j++) {
if (a[j] > a[i]) {
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
printf("Printing Sorted Element List ...\n");
for (i = 0; i < 10; i++) {
printf("%d\n", a[i]);
}
}
This snippet demonstrates how swapping can be used to sort an array efficiently.
Finding the Largest and Second Largest Elements
Sometimes, you might need to identify the top values in an array. The following program reads user input into an array and then finds the largest and second largest numbers:
#include <stdio.h>
void main() {
int arr[100], n, i, largest, sec_largest;
printf("Enter the size of the array: ");
scanf("%d", &n);
printf("Enter the elements of the array: ");
for(i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
largest = arr[0];
sec_largest = arr[1];
for(i = 0; i < n; i++) {
if(arr[i] > largest) {
sec_largest = largest;
largest = arr[i];
} else if(arr[i] > sec_largest && arr[i] != largest) {
sec_largest = arr[i];
}
}
printf("Largest = %d, Second Largest = %d", largest, sec_largest);
}
This example showcases reading input, comparing elements, and updating the largest values as the program iterates through the array.
What's Your Reaction?
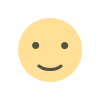
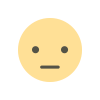
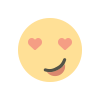
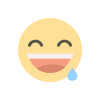
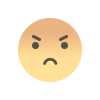
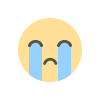
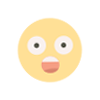