Shortcut Operator in C
In C programming, there are several shortcut operators that allow performing operations and assignment in a concise way. These operators combine an operation with assignment, making code more efficient and readable. When using these operators, the left-hand operand is updated based on the right-hand expression, reducing the need for explicit repetition.
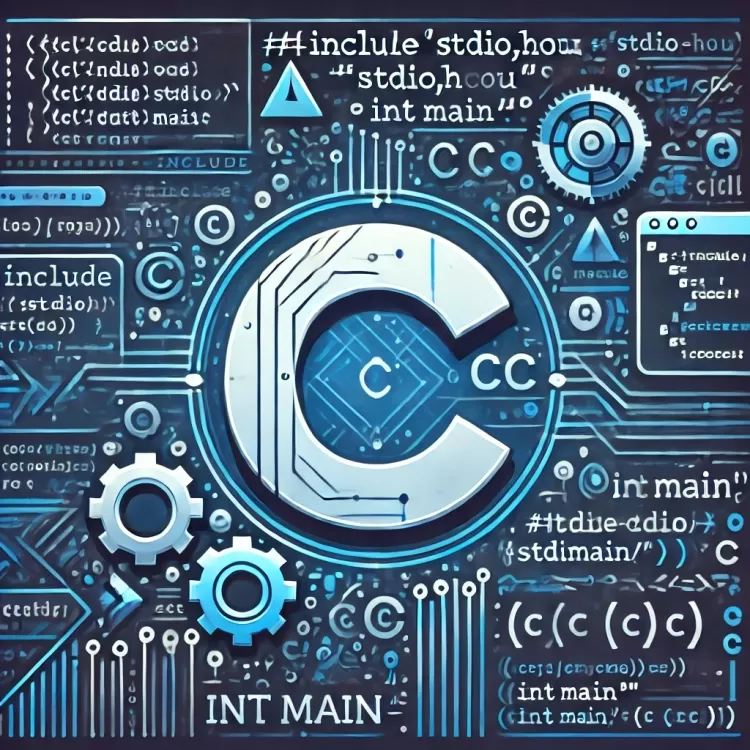
Common Shortcut Operators:
Operator |
Description |
+= |
Adds the right operand to the left operand and assigns the result to the left operand. |
-= |
Subtracts the right operand from the left operand and assigns the result to the left operand. |
*= |
Multiplies the left operand by the right operand and assigns the result to the left operand. |
/= |
Divides the left operand by the right operand and assigns the result to the left operand. |
%= |
Takes the modulus (remainder) of the left operand divided by the right operand and assigns the result to the left operand. |
Examples and Usage:
1.Addition:
int x = 5;
x += 3; // Equivalent to: x = x + 3;
printf("%d", x); // Output: 8
2.Subtraction
int x = 10;
x -= 4; // Equivalent to: x = x - 4;
printf("%d", x); // Output: 6
3.Multiplication
int x = 7;
x *= 2; // Equivalent to: x = x * 2;
printf("%d", x); // Output: 14
4.Division
int x = 20;
x /= 5; // Equivalent to: x = x / 5;
printf("%d", x); // Output: 4
5.Modulus
int x = 17;
x %= 3; // Equivalent to: x = x % 3;
printf("%d", x); // Output: 2
Advantages of Using Shortcut Operators
- Reduces redundancy in expressions.
- Improves code readability and maintainability.
- Often results in more efficient execution by avoiding repeated variable lookups.
Practice Questions
1. x-=y+1 is same as:
- x=y-1;
- x=x-y-1;
- compiler dependent
- none
What's Your Reaction?
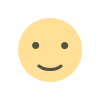
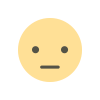
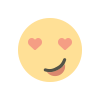
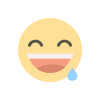
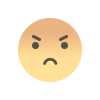
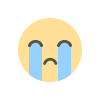
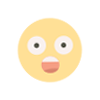