Relational Operators in C programming
Relational operators are used to compare two values. The result of the relational operator is either "1" or "0" these operators are commonly used in decision making statements such as
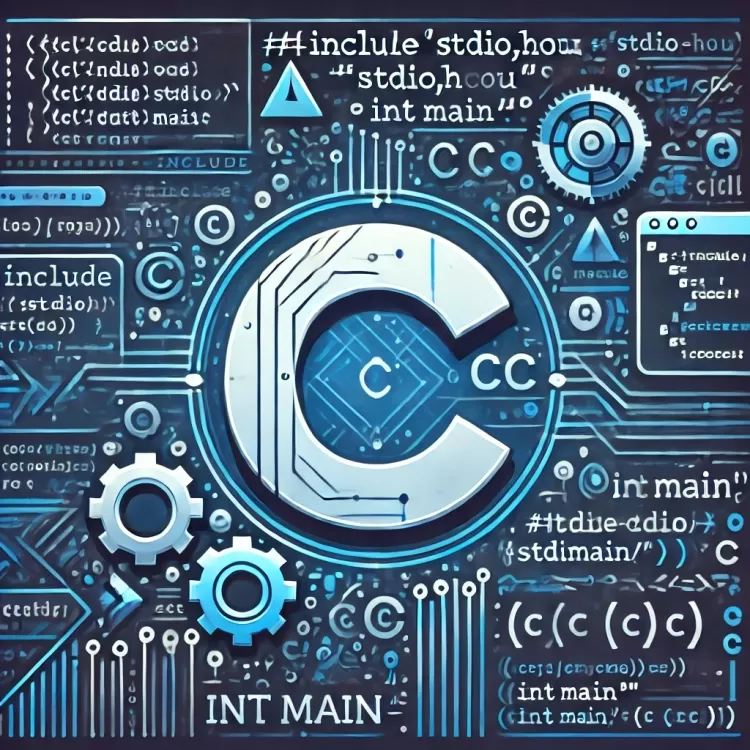
"if" , "while", "for" and "do-while" loops.
here is the list of Relational operators:
Operator |
Description |
Example |
Result |
== |
Equal to |
a == b |
1 if equal, 0 otherwise |
!= |
Not equal to |
a != b |
1 if not equal, 0 otherwise |
> |
Greater than |
a > b |
1 if a is greater, 0 otherwise |
< |
Less than |
a < b |
1 if a is smaller, 0 otherwise |
>= |
Greater than or equal to |
a >= b |
1 if a is greater or equal, 0 otherwise |
<= |
Less than or equal to |
a <= b |
1 if a is smaller or equal, 0 otherwise |
simple example:
#include
int main() {
int a = 10, b = 20;
if (a == b) {
printf("a is equal to b\n");
} else {
printf("a is not equal to b\n");
}
if (a < b) {
printf("a is less than b\n");
}
if (a > b) {
printf("a is greater than b\n");
} else {
printf("a is not greater than b\n");
}
return 0;
}
Output:
Precedence:
practice questions:
1. if int a=5, b=2, c=1,d; then the expression d=a>b>c; what is the value of d?
2. if int a=5, b=2,c=1,d; the what is value of d? if the expression is d=c+a>b;
Relational Operators Precedence and Associativity:
Operator |
Description |
Precedence Level |
Associativity |
== |
Equal to |
Lower |
Left-to-right |
!= |
Not equal to |
Lower |
Left-to-right |
> |
Greater than |
Higher |
Left-to-right |
< |
Less than |
Higher |
Left-to-right |
>= |
Greater than or equal to |
Higher |
Left-to-right |
<= |
Less than or equal to |
Higher |
Left-to-right |
3.evaluate the expression x=1<0==0;
What's Your Reaction?
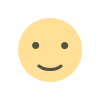
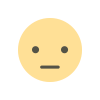
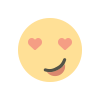
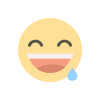
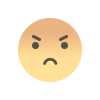
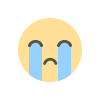
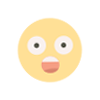