Top Core Java Concepts In Detail
core Java concepts are fundamental for developing robust and scalable applications.
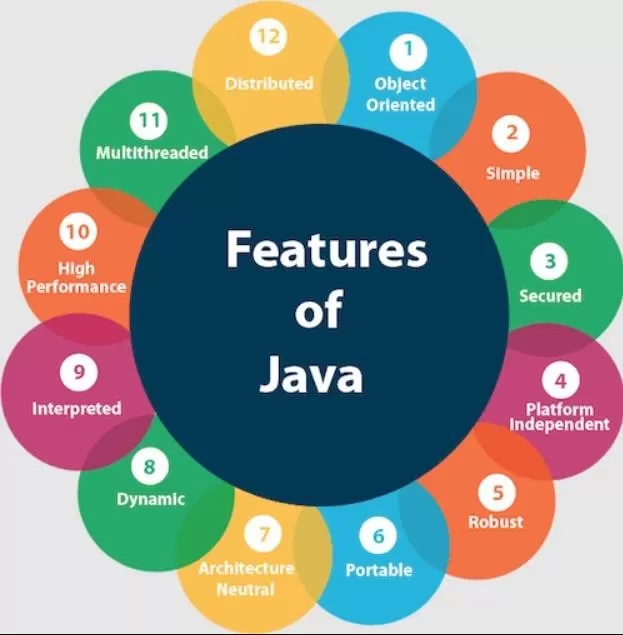
1.Object-Oriented Programming (OOP) Principles:
Java is fundamentally an object-oriented programming language that leverages key OOP concepts:
- Class and Object: A class serves as a blueprint for creating objects. It combines data and methods to manipulate that data. An object is an instance of a class.
- Inheritance: This allows a subclass to inherit attributes and methods from a superclass, fostering code reuse and a natural hierarchy.
- Polymorphism: This enables methods to perform different tasks based on the context. It includes compile-time (method overloading) and runtime (method overriding) polymorphism.
- Encapsulation: This involves wrapping data (variables) and code (methods) into a single unit, restricting direct access to some of an object's components. Access modifiers (private, public, protected) typically achieve this.
- Abstraction: This focuses on hiding complex implementation details and exposing only essential features, achieved through abstract classes and interfaces.
2. Data Types and Variables:
Java supports a variety of data types:
- Primitive Data Types: These include byte, short, int, long (for integers), float, double (for floating-point numbers), char (for characters), and boolean (for true/false values).
- Non-Primitive Data Types: These encompass classes, arrays, and interfaces.
3.Variables in Java are categorized based on their scope:
- Instance Variables: Defined within a class but outside any method. These variables are unique to each class instance.
- Class Variables (Static Variables): Defined with the static keyword and shared among all class instances.
- Local Variables: Defined within methods, constructors, or blocks, limited to the scope in which they’re declared.z
4. Control Flow Statements:
Java offers various control flow statements to manage program execution:
- If-Else: Conditional statements that execute code blocks based on a condition.
- Switch: A control statement allowing variable testing against a list of values.
- For, While, and Do-While Loops: These enable iteration, allowing code blocks to repeat based on a condition.
5. Arrays:
Arrays in Java store multiple values in a single variable. They can be single-dimensional or multi-dimensional, with elements indexed from 0.
6. Exception Handling:
Java handles runtime errors through exception handling using five keywords: try, catch, finally, throw, and throws.
- Try: Contains code that may throw an exception.
- Catch: Catches and handles exceptions.
- Finally: Executes code after try and catch, regardless of an exception.
- Throw: Explicitly throws an exception.
- Throws: Indicates exceptions that a method might throw.
7. Collections Framework:
The Collections Framework offers interfaces and classes for managing a group of objects. Key interfaces include:
- List: An ordered collection permitting duplicate elements (e.g., ArrayList, LinkedList).
- Set: A collection prohibiting duplicate elements (e.g., HashSet, TreeSet).
- Map: Maps keys to values without duplicate keys (e.g., HashMap, TreeMap).
8. Multithreading:
Java’s multithreading allows concurrent execution of multiple threads to optimize CPU usage. It supports this through the Thread class and Runnable interface.
9. Input and Output (I/O) Operations:
Java I/O handles reading and writing data sources (e.g., files, networks). Key classes include FileReader, FileWriter, BufferedReader, and BufferedWriter.
10. Java APIs:
Java provides a vast library of prewritten classes (Java API) for building applications, including data structures, networking, and file I/O.
These core Java concepts are fundamental for developing robust and scalable applications.
What's Your Reaction?
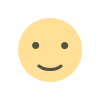
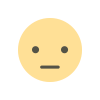
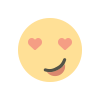
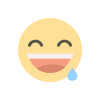
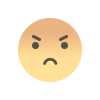
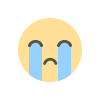
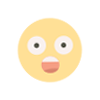