Java Map Interface: An Essential Tool for Efficient Data Handling
The "Map interface" in Java, part of the Java Collections Framework, facilitates the storage of key-value pairs. Unlike other collection types like lists and sets, maps allow for the association of a value with a specific key, enabling the efficient retrieval of values based on their corresponding keys. This capability makes maps a powerful tool for managing data that requires quick lookups, updates, and organization.
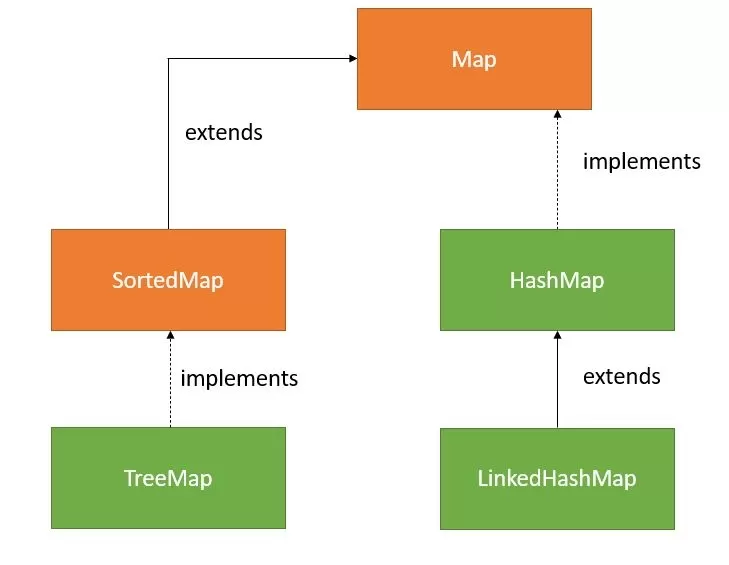
Key Characteristics:
1. Key Value Pairs : Maps store data as key value pairs, where each key is unique and maps to a specific value, enabling efficient retrieval using the associated keys.
2. Unique Keys : Each key in a map is unique, meaning no two keys are identical. However, multiple keys can be associated with the same value.
3. Null Values : Depending on the implementation, maps can allow null keys and values. For example, 'HashMap' permits one null key and multiple null values, whereas 'TreeMap' does not allow null keys but allows null values.
Common Implementations:
Several concrete classes implement the Map interface, each offering unique performance characteristics and use cases. Commonly used implementations include:
1. HashMap :
Characteristics : Uses a hash table for implementation, providing constant time performance for basic operations like 'get' and 'put', assuming the hash function disperses elements properly.
Usage : Ideal for general purpose use when fast retrieval and insertion are required. Allows one null key and multiple null values.
Example :
Map<String, Integer> hashMap = new HashMap<>();
hashMap.put("Apple", 1);
hashMap.put("Banana", 2);
hashMap.put("Cherry", 3);
2. LinkedHashMap :
Characteristics : Extends 'HashMap' and maintains a doubly linked list through all its entries, providing a predictable iteration order (the order in which keys were inserted).
Usage : Suitable for scenarios where iteration order matters.
Example :
Map<String, Integer> linkedHashMap = new LinkedHashMap<>();
linkedHashMap.put("Apple", 1);
linkedHashMap.put("Banana", 2);
linkedHashMap.put("Cherry", 3);
3. TreeMap :
Characteristics : Uses a red black tree for implementation, ordering its keys based on their natural ordering or a specified comparator. Provides guaranteed log(n) time cost for basic operations like 'get' and 'put'.
Usage : Ideal for situations requiring a sorted order of keys.
Example :
Map<String, Integer> treeMap = new TreeMap<>();
treeMap.put("Apple", 1);
treeMap.put("Banana", 2);
treeMap.put("Cherry", 3);
4. Hashtable :
Characteristics : Uses a synchronized hash table for implementation. It does not allow null keys or values and is generally slower than 'HashMap' due to synchronization overhead.
Usage : Suitable for scenarios requiring thread safe operations.
Example :
Map<String, Integer> hashtable = new Hashtable<>();
hashtable.put("Apple", 1);
hashtable.put("Banana", 2);
hashtable.put("Cherry", 3);
Important Methods:
The Map interface provides several methods for manipulating and interacting with the key value pairs stored in a map. Key methods include:
put(K key, V value) : Associates the specified value with the specified key in the map.
map.put("Mango", 4);
get(Object key) : Returns the value to which the specified key is mapped, or 'null' if the map contains no mapping for the key.
Integer value = map.get("Banana");
remove(Object key) : Removes the mapping for the specified key from the map if present.
map.remove("Apple");
containsKey(Object key) : Returns 'true' if the map contains a mapping for the specified key.
boolean hasKey = map.containsKey("Cherry");
containsValue(Object value) : Returns 'true' if the map maps one or more keys to the specified value.
boolean hasValue = map.containsValue(3);
keySet() : Returns a 'Set' view of the keys contained in the map.
Set<String> keys = map.keySet();
values() : Returns a 'Collection' view of the values contained in the map.
Collection<Integer> values = map.values();
entrySet() : Returns a 'Set' view of the mappings contained in the map.
Set<Map.Entry<String, Integer>> entries = map.entrySet();
Usage Scenarios:
1. Database Cache : Maps can implement caches for database records, where keys are record IDs, and values are the records themselves. 'HashMap' or 'LinkedHashMap' are commonly used for this purpose.
Map<Integer, Record> recordCache = new HashMap<>();
2. Configuration Properties : Maps often store configuration properties, with property names as keys and property values as values.
Map<String, String> config = new HashMap<>();
config.put("url", "https://smartlocus.com");
config.put("timeout", "5000");
3. Frequency Counting : Maps are useful for frequency counting in data processing tasks, where keys are items and values are their counts.
Map<String, Integer> frequencyMap = new HashMap<>();
frequencyMap.put("Apple", 5);
frequencyMap.put("Banana", 3);
4. Graph Representation : Maps effectively represent graphs, where keys are node identifiers and values are lists of adjacent nodes.
Map<String, List<String>> graph = new HashMap<>();
graph.put("A", Arrays.asList("B", "C"));
graph.put("B", Arrays.asList("A", "D"));
The Map interface in Java is a versatile and powerful tool for managing key value pairs efficiently. With various implementations such as 'HashMap', 'LinkedHashMap', 'TreeMap', and 'Hashtable', developers can choose the most suitable map based on their specific requirements, whether it's for performance, maintaining order, or ensuring thread safety. The methods provided by the Map interface allow for efficient manipulation and retrieval of key value pairs, making it an essential component of the Java Collections Framework for handling data that requires quick lookups and updates.
What's Your Reaction?
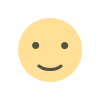
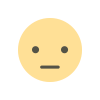
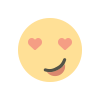
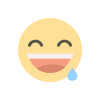
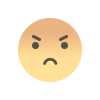
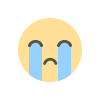
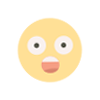