Java Collections Framework: Key Components and Benefits
The Collections Framework in Java is a robust and versatile toolkit that simplifies the management of groups of objects. It offers a standardized set of interfaces, implementations, and algorithms, enabling developers to work with collections efficiently and effectively. Whether for storing data, implementing caching mechanisms, or performing complex manipulations, the Collections Framework provides the necessary tools to achieve these objectives with ease.
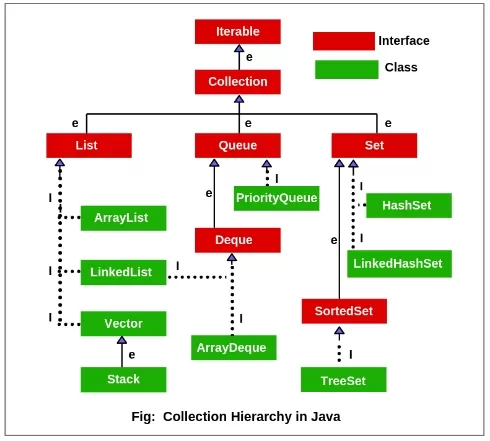
The Collections Framework in Java offers a cohesive architecture for representing and manipulating groups of objects. Essential to the Java Standard Edition, it provides a range of interfaces, implementations, and algorithms for handling various collections such as lists, sets, and maps. Since its introduction in Java 2 (Java 1.2), it has become a fundamental part of the language, providing a standardized way to manage collections.
Key Components of the Collections Framework
-
Interfaces: The framework defines multiple interfaces representing different types of collections, including:
-
Collection: The root interface that represents a group of objects.
-
List: An ordered collection permitting duplicates. Examples are ArrayList, LinkedList, and Vector.
-
Set: A collection that prohibits duplicates. Examples include HashSet, LinkedHashSet, and TreeSet.
-
Queue: A collection for holding elements prior to processing. Examples are PriorityQueue and LinkedList.
-
Map: An object that maps keys to values, with no duplicate keys allowed. Examples include HashMap, TreeMap, and LinkedHashMap.
-
-
Implementations: These are the concrete classes that implement the collection interfaces, each with unique performance characteristics and use cases. Common implementations include:
-
ArrayList: A resizable array implementation of the List interface, offering fast random access.
-
LinkedList: A doubly-linked list implementing List and Deque interfaces, facilitating quick insertions and deletions.
-
HashSet: A hash table-backed Set implementation, providing constant-time basic operations.
-
TreeSet: A sorted set based on a red-black tree, offering logarithmic time operations.
-
HashMap: A hash table-backed Map implementation, also offering constant-time operations.
-
TreeMap: A sorted map based on a red-black tree, with logarithmic time operations.
-
-
Algorithms: The framework includes algorithms for collections, such as searching, sorting, and shuffling, implemented as static methods in the Collections utility class. Common algorithms are:
-
sort: Sorts a list in ascending order.
-
binarySearch: Searches for an element in a sorted list using binary search.
-
shuffle: Randomly rearranges the elements in a list.
-
reverse: Reverses the order of elements in a list.
-
min and max: Finds the smallest and largest elements in a collection.
-
Advantages of Using the Collections Framework
-
Unified Architecture: Provides a standard approach to managing collections, making it easier for developers to learn and use.
-
Reusability: Offers reusable data structures and algorithms, reducing the need for custom implementations.
-
Performance: Various implementations are optimized for different scenarios, allowing developers to select the most appropriate collection.
-
Interoperability: Ensures seamless interaction between collections due to consistent interface hierarchy.
-
Thread Safety: Includes synchronized implementations like Vector and Hashtable, with utility methods in Collections for creating synchronized collections.
-
Generics: Introduced in Java 5, generics provide type safety by allowing collections to be parameterized with specific types, minimizing runtime errors.
Common Usage Scenarios
-
Storing and Manipulating Data: Collections are widely used to store and manipulate objects in applications, such as using ArrayList to store items in a shopping cart.
-
Searching and Sorting: Algorithms like binarySearch and sort are commonly used for searching and sorting elements.
-
Caching: Collections like HashMap and LinkedHashMap are often used to implement caching mechanisms for quick key-value retrieval.
-
Event Handling: Collections like LinkedList are used to manage lists of event listeners in graphical user interfaces.
-
Data Structures: Specialized collections like PriorityQueue and TreeSet are used to implement advanced data structures like priority queues and sorted sets.
What's Your Reaction?
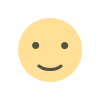
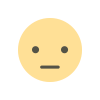
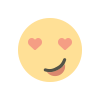
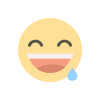
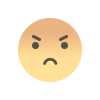
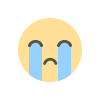
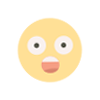