Java Set Interface: Ensuring Performance, Order, and Uniqueness
The Set interface in Java is a critical component of the Java Collections Framework, providing an efficient way to manage collections of unique elements. With implementations like HashSet, LinkedHashSet, and TreeSet, developers can choose the most suitable set based on their specific requirements, whether it’s for performance, maintaining order, or sorting elements. The Set interface’s methods ensure efficient operations, making it an essential tool for managing collections without duplicates.
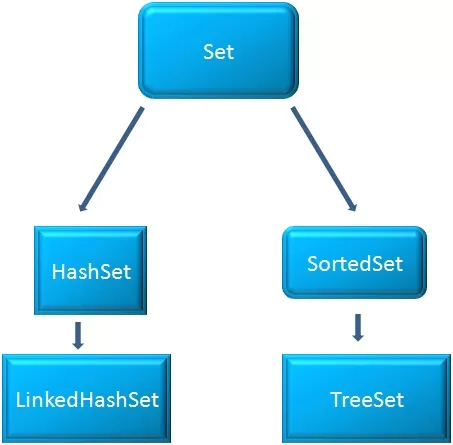
The "Set interface" in Java is an integral part of the Java Collections Framework, designed to represent a collection that prohibits duplicate elements. It embodies the mathematical set abstraction and includes methods for manipulating these sets. As a subinterface of the Collection interface, it plays a vital role in managing unique elements.
Key Characteristics:
1. No Duplicates : Sets do not allow duplicate elements. If an element already exists in the set, adding it again does not change the set.
2. Unordered : Most Set implementations do not maintain a specific order of elements. The order can vary depending on the implementation.
Common Implementations:
Several concrete classes implement the Set interface, each with distinct performance characteristics and use cases. Some widely used implementations include:
1. HashSet :
Characteristics : Implements the Set interface using a hash table. It offers constant time performance for basic operations like add, remove, and contains, assuming the hash function distributes elements properly.
Usage : Ideal for situations where performance is crucial and the order of elements is not important.
Example :
Set
2. LinkedHashSet :
Characteristics : Extends HashSet and maintains a doubly linked list through its entries, providing a predictable iteration order (insertion order).
Usage : Useful when it is necessary to maintain the iteration order of elements.
Example :
Set
3. TreeSet :
Important Methods:
The Set interface includes several methods for manipulating and interacting with elements. Some key methods are:
- add(E e) : Adds the specified element to the set if it is not already present.
set.add("Mango");
- remove(Object o) : Removes the specified element from the set if it is present.
set.remove("Apple");
- contains(Object o) : Checks if the set contains the specified element.
boolean contains = set.contains("Cherry");
- size() : Returns the number of elements in the set.
int size = set.size();
- isEmpty() : Checks if the set contains no elements.
boolean isEmpty = set.isEmpty();
- clear() : Removes all elements from the set.
set.clear();
Usage Scenarios:
1. Storing Unique Elements : Sets are ideal for storing unique elements where duplicates are not permitted, such as storing unique user IDs.
Set
2. Maintaining Order : When maintaining the order of elements is necessary, LinkedHashSet can be used.
Set
3. Sorted Elements : TreeSet is the appropriate choice when a sorted collection is required.
Set
The Set interface in Java is an essential part of the Java Collections Framework, offering an efficient solution for managing collections of unique elements. Developers can select from various implementations like HashSet, LinkedHashSet, and TreeSet, depending on their specific needs, whether it's optimizing for performance, preserving order, or ensuring sorted elements. The methods provided by the Set interface guarantee efficient operations, making it indispensable for handling collections without duplicates.
What's Your Reaction?
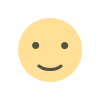
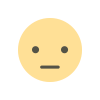
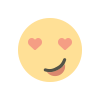
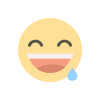
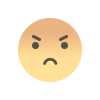
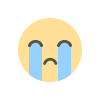
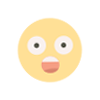