Exploring Java 8 Features: A Game Changer for Developers
Java 8, released in March 2014, brought revolutionary enhancements to the Java programming language, significantly improving performance, readability, and maintainability. It introduced functional programming, improved collections processing, new APIs, and better concurrency support. In this post, we’ll explore the most important Java 8 features that every developer should know.
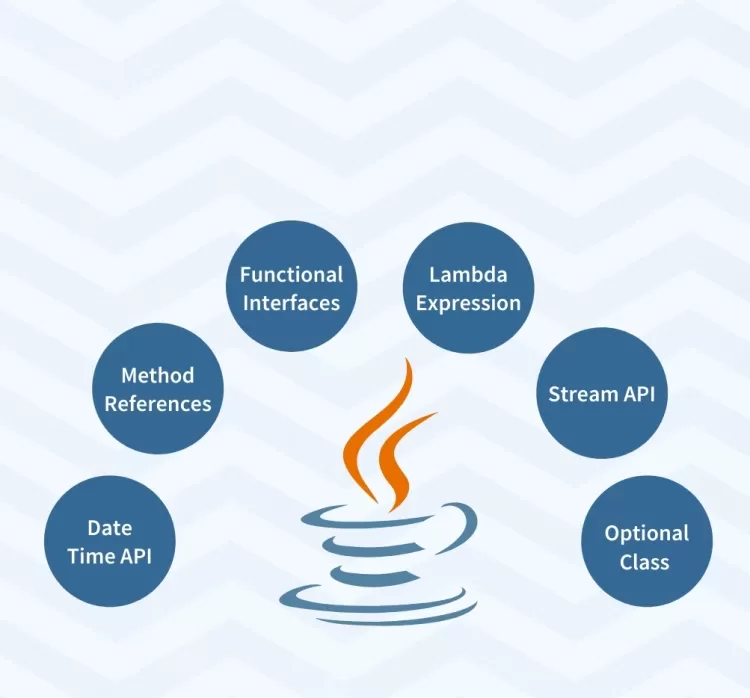
1. Lambda Expressions
Lambda expressions enable functional programming in Java, making the code more concise and readable. They help reduce boilerplate code and enhance efficiency when handling collections.
Example:
List
names = Arrays.asList("Alice", "Bob", "Charlie"); names.forEach(name -> System.out.println(name));
Here, name -> System.out.println(name) is a lambda expression that simplifies the traditional anonymous inner class implementation.
2. Functional Interfaces
A functional interface contains only one abstract method, allowing the use of lambda expressions. Java 8 introduces several built-in functional interfaces, including Predicate, Function, and Consumer.
Example:
@FunctionalInterfaceinterface
MyFunctionalInterface{ void execute(); } public class
LambdaExample{ public static void
main(String[]args) { MyFunctionalInterface func = () -> System.out.println("Hello from Lambda!"); func.execute();
}}
3. Stream API
The Stream API facilitates functional-style operations on collections, enhancing efficiency and readability in data processing. It supports operations such as filtering, mapping, and reducing.
Example:
List
.filter(nnumbers = Arrays.asList(1, 2, 3, 4, 5); numbers.stream() -> n % 2 == 0) .forEach(System.out::println);
This filters out even numbers from the list and prints them.
4. Default Methods in Interfaces
Java 8 allows interfaces to have default methods with implementations, ensuring backward compatibility without breaking existing code.
Example:
interface Vehicle { default void start() { System.out.println("Vehicle is starting...");
} }class Car implements Vehicle {} public class Test { public static void main(String[] args) { Car car = new Car(); car.start(); } }
Here, Car inherits the start() method from the Vehicle interface.
5. Optional Class
The Optional class helps prevent NullPointerException by providing a container object that may or may not contain a non-null value.
Example:
Optional
Optional.ofNullable(null);optional = System.out.println(optional.orElse("Default Value"));
This prints "Default Value" since the optional variable is empty.
6. Method References
Method references improve lambda expressions’ readability by directly referring to existing methods.
Example:
List names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
Here, System.out::println is a method reference that replaces name -> System.out.println(name).
7. New Date and Time API
Java 8 introduced the java.time package, offering improved methods for handling date and time operations.
Example:
LocalDate today = LocalDate.now();
System.out.println("Today's Date: " + today);
This prints the current date.
8. Collectors Class
The Collectors utility class aids in processing stream data into collections or performing statistical operations.
Example:
List names = Arrays.asList("Alice", "Bob", "Charlie");
String result = names.stream().collect(Collectors.joining(", "));
System.out.println(result);
This joins the list elements into a single string: "Alice, Bob, Charlie".
9. Parallel Streams
Parallel streams enable multi-threaded execution of collection operations, boosting performance on large datasets.
Example:
List
Arrays.asList(1,numbers = 2, 3, 4, 5); numbers.parallelStream().forEach(System.out::println);
This processes elements in parallel, enhancing execution speed.
10. Base64 Encoding and Decoding
Java 8 provides a built-in Base64 API for encoding and decoding data.
Example:
String encoded = Base64.getEncoder().encodeToString("Hello, Java 8".getBytes());
System.out.println("Encoded: "+ encoded);
This prints the Base64-encoded version of the string.
Java 8 introduced a range of powerful features that enhance the flexibility, readability, and performance of Java applications. By leveraging lambda expressions, the Stream API, default methods, and the new Date-Time API, developers can write cleaner and more efficient code.
What's Your Reaction?
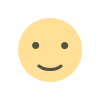
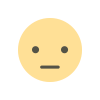
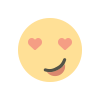
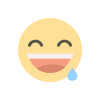
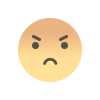
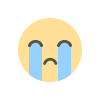
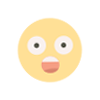